Run code in background using PHP-FPM
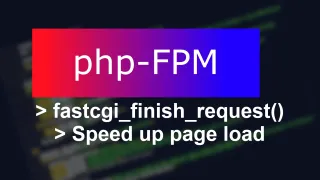
After a user have filled out a form or maybe you are doing some statistic, you often don't want the user to wait for this to run and there's options to run it in background
PHP-FPM
Pre-requisites
If you're using PHP on Nginx, you already have PHP-FPM and you're good to go. If you're running Apache, you'll need to add PHP-FPM or this approach won't work
Setup
Most of your code is probably fairly quick, until you get to the backend stuff, which can take up to multiple minutes depending on size of data to be processed. You probably don't wish that the user have to wait for it to finish processing, but at the same time needs this data to actually be processed
[snippet]#Example code
<HTML>
//Do stuff for user
...
</HTML>
<?php
//Backend stuff, such as statics or updating database with form input
... time consuming jobs ...
//Will be printed on screen for user to see
echo "Backend stuff is completed";
?>[/snippet]
This is where fastcgi_finish_request() comes in play. This will stop the output to user display when triggered, so that the page is done loading at this time for user, making the page load quicker. While continuing processing remaining code on the server, no longer affected by users browser session (such as reloads, moving to another page or leaving your site)
[snippet]#Example code
<HTML>
//Do stuff for user
...
</HTML>
<?php
// Run remaining code in background
fastcgi_finish_request();
// Page have finished loading for user
// Below code will now be run on server side, not affected by user navigation
// Backend stuff, such as statics or updating database with form input
... time consuming jobs ...
//Will NOT be printed on screen
echo "Backend stuff is completed";
?>[/snippet]
Pitfalls
PHP Workers
fastcgi_finish_request() works well to speed up the current session, but it will consume a PHP worker until it's done processing the backend stuff. The general recommendation is to use this feature sparingly, especially on sites with heavy traffic. As excessive use, can consume up all the PHP workers and users will face slowness, while they wait for a PHP worker to be available
Output to screen
After fastcgi_finish_request() is called, nothing more will be output to the screen for user to see. So it's important it's called after everything that's needed for user to see is run or user won't see it
Output to other areas, such as email, can still be triggered and will work fine
Performance improvement
How much performance improvement will be gained, depends on what's being run after fastcgi_finish_request() is called and how demanding it is
After we implemented it, we saw an average page load reduction of 2 seconds per page. As the jobs doing statistics and similar tasks are run after the page is rendered for user and user don't need to wait for it to run
Tags: #NGINX #PHP #RunInBackgroundPHP #phpfpm